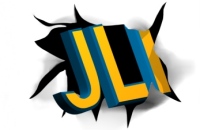 |
JLI Spieleprogrammierung
|
Vorheriges Thema anzeigen :: Nächstes Thema anzeigen |
Autor |
Nachricht |
PeaceKiller JLI Master

Alter: 35 Anmeldedatum: 28.11.2002 Beiträge: 970
Medaillen: Keine
|
Verfasst am: 19.01.2006, 20:58 Titel: Show me your code and I will tell you who you are. #2 |
|
|
Es gibt eine neue Runde!
Selbe Regeln wie letztes mal, also:
Jeder der selber mitmacht muss zwei Codes unkommentiert lassen.
Mitmachen tun:
bld, Christian Rousselle, Clythoss, Dragon, Fallen, Fast Hawk, Kronos, LordHoto, Oliver
CPP: | #ifndef _TEXTURE_
#define _TEXTURE_
// Includes ///////////////////////////////////////////////////////////////////
#include <gl/gl.h>
// Namensbereich //////////////////////////////////////////////////////////////
namespace video
{
// Vorw�tsdeklarationen //////////////////////////////////////////////////////
class TextureManager;
// Klassen ////////////////////////////////////////////////////////////////////
class Texture
{
public:
Texture(unsigned int id, unsigned long w, unsigned long h)
: id_(id)
, width_(w)
, height_(h)
{
}
~Texture()
{
}
inline unsigned long getWidth() const{return width_;}
inline unsigned long getHeight() const{return height_;}
void bind1d() const {glBindTexture(GL_TEXTURE_1D, id_); }
void bind2d() const {glBindTexture(GL_TEXTURE_2D, id_); }
friend class TextureManager;
private:
unsigned long width_;
unsigned long height_;
unsigned int id_;
};
}
#endif
|
CPP: | #include <windows.h>
#include "exception.h"
Exception::Exception(const TCHAR* text, const TCHAR* source, int line)
{
wsprintf(description,
TEXT("Interner Fehler festgestellt!\n\n")
TEXT("BESCHREIBUNG:\n%s\n\n")
TEXT("QUELLCODEDATEI: %s \n")
TEXT("ZEILE: %d"),
text, source, line);
}
Exception::Exception(const TCHAR* text, const TCHAR* file, const TCHAR* source, int line)
{
wsprintf(description,
TEXT("Interner Fehler festgestellt!\n\n")
TEXT("BESCHREIBUNG:\n[%s]%s\n\n")
TEXT("QUELLCODEDATEI: %s \n")
TEXT("ZEILE: %d"),
file, text, source, line);
}
|
CPP: | #ifndef CSMANAGER_H_
#define CSMANAGER_H_
#include "Singleton.h"
enum CSI
{
CSI_TEXTCTRLTEXTCHANGE = 0,
};
class CSManager : public Singleton<CSManager>
{
static const unsigned COUNT = 64;
bool registers[COUNT];
public:
CSManager();
void TryEnter(const CSI id)
{
while(registers[id]);
registers[id] = 1;
}
void Leave(const CSI id)
{
registers[id] = 0;
}
};
#endif |
CPP: | class Button
{
public:
Button(void);
~Button(void);
bool UpdateButton(dims2 MouseState);
Button operator=(Button& lpButton);
Button & operator+=(Button& lpButton){return *this;}
Button & operator-=(Button& lpButton){return *this;}
Button & operator*=(Button& lpButton){return *this;}
Button & operator/=(Button& lpButton){return *this;}
basic_string<wchar_t> Value;
basic_string<wchar_t> TexturKey;
basic_string<wchar_t> TexturKeyHover;
basic_string<wchar_t> TexturKeyOnClick;
basic_string<wchar_t> TexturKeyVisited;
bool Hover;
bool OnClick;
bool Visited;
rect Position;
};
Button::Button(void)
{
Hover = FALSE;
Visited = FALSE;
OnClick = FALSE;
}
Button::~Button(void)
{
}
bool Button::UpdateButton(dims2 MouseState)
{
if((!(MouseState.rgbButtons[0] == 0x80)) && OnClick == TRUE)
{
if(CursorPosition.x > Position.left && CursorPosition.x < Position.right &&
CursorPosition.y > Position.top && CursorPosition.y < Position.bottom)
{
Visited = TRUE;
OnClick = FALSE;
return TRUE;
}
else
{
OnClick = FALSE;
}
}
if(CursorPosition.x > Position.left && CursorPosition.x < Position.right &&
CursorPosition.y > Position.top && CursorPosition.y < Position.bottom && OnClick == FALSE)
{
Hover = TRUE;
if((!(MouseState.rgbButtons[0] == 0x80)) && OnClick == TRUE)
{
OnClick=TRUE;
}
}
else
{
Hover = FALSE;
}
return FALSE;
}
Button Button::operator =(Button& lpButton)
{
Value = lpButton.Value;
TexturKey = lpButton.TexturKey;
TexturKeyHover = lpButton.TexturKeyHover;
TexturKeyOnClick = lpButton.TexturKeyOnClick;
TexturKeyVisited = lpButton.TexturKeyVisited;
Hover = lpButton.Hover;
OnClick = lpButton.OnClick;
Visited = lpButton.Visited;
Position = lpButton.Position;
return *this;
} |
CPP: | void ThemeClassic::drawCheckbox(const Common::Rect &r, const Common::String &str, bool checked, kState state = kStateEnabled) {
if (!_initOk)
return;
Common::Rect r2 = r;
int checkBoxSize = getFontHeight();
if (checkBoxSize > r.height()) {
checkBoxSize = r.height();
}
_screen.fillRect(r, _bgcolor);
box(r.left, r.top, checkBoxSize, checkBoxSize, _color, _shadowcolor);
if (checked) {
// TODO: implement old style
r2.top += 2;
r2.bottom = r.top + checkBoxSize - 2;
r2.left += 2;
r2.right = r.left + checkBoxSize - 2;
_screen.fillRect(r2, getColor(state));
r2 = r;
}
r2.left += checkBoxSize + 10;
r2.top += 3;
_font->drawString(&_screen, str, r2.left, r2.top, r2.width(), getColor(state), Graphics::kTextAlignCenter, 0, true);
addDirtyRect(r);
} |
CPP: | void CSceneNodeMesh::SetMeshID(int ID)
{
m_MeshID = ID;
CXFileMesh* XFileMesh = CGlobals::Get().m_ResourceManager.GetMesh(m_MeshID);
const D3DXVECTOR3* pMax = XFileMesh->GetBoundingVolume()->GetMax();
const D3DXVECTOR3* pMin = XFileMesh->GetBoundingVolume()->GetMin();
NxBoxShapeDesc BoxDesc;
BoxDesc.dimensions = static_cast<NxVec3>(((*pMax) - (*pMin)) / 2.0f);
NxBodyDesc BodyDesc;
BodyDesc.angularDamping = 0.5f;
NxActorDesc ActorDesc;
ActorDesc.shapes.pushBack(&BoxDesc);
ActorDesc.body = &BodyDesc;
ActorDesc.density = 1.0f;
m_pActor = m_pScene->GetPhysScene()->createActor(ActorDesc);
}
void CSceneNodeMesh::SetJoint(const char* With)
{
CSceneNode* pNode = m_pScene->FindNode(With);
if(!pNode)
{
return;
}
NxFixedJointDesc FixedJointDesc;
FixedJointDesc.actor[0] = m_pActor;
FixedJointDesc.actor[1] = pNode->GetActor();
NxVec3 Pos(0.0f, 5.0f, 0.0f);
FixedJointDesc.setGlobalAnchor(Pos);
NxJoint* pJoint = m_pScene->GetPhysScene()->createJoint(FixedJointDesc);
pJoint->setBreakable(20.0f, 20.0f);
}
void CSceneNodeMesh::SetPosition(float x, float y, float z)
{
m_pActor->setGlobalPosition(NxVec3(x, y, z));
}
void CSceneNodeMesh::Render()
{
m_pActor->getGlobalPose().getColumnMajor44(reinterpret_cast<NxF32*>(&m_matWorld));
CGlobals::Get().m_pd3dDevice->SetTransform(D3DTS_WORLD, &m_matWorld);
CGlobals::Get().m_ResourceManager.GetMesh(m_MeshID)->Render(m_bSelected);
}
bool CSceneNodeMesh::HitTest(const D3DXVECTOR3* pPickVec, const D3DXMATRIX* pViewMatrix, D3DXVECTOR3* pHitVec)
{
D3DXVECTOR3 vPickRayDir;
D3DXVECTOR3 vPickRayOrig;
// inverse Matrix der zusammengesetzen WorldView Matrix berechnen
D3DXMATRIXA16 m;
m = m_matWorld * (*pViewMatrix);
D3DXMatrixInverse( &m, NULL, &m );
// Picking Ray vom ScreenSpace in WorldSpace umsetzen
vPickRayDir.x = pPickVec->x * m._11 + pPickVec->y * m._21 + pPickVec->z * m._31;
vPickRayDir.y = pPickVec->x * m._12 + pPickVec->y * m._22 + pPickVec->z * m._32;
vPickRayDir.z = pPickVec->x * m._13 + pPickVec->y * m._23 + pPickVec->z * m._33;
vPickRayOrig.x = m._41;
vPickRayOrig.y = m._42;
vPickRayOrig.z = m._43;
CXFileMesh* XFileMesh = CGlobals::Get().m_ResourceManager.GetMesh(m_MeshID);
return 0 != (D3DXSphereBoundProbe(XFileMesh->GetBoundingVolume()->GetCenter(),
XFileMesh->GetBoundingVolume()->GetRadius(),
&vPickRayOrig, &vPickRayDir));
}
|
Die Codes sind komplett zufällig gewählt. Viel Spaß beim raten! _________________ »If the automobile had followed the same development cycle as the computer, a Rolls-Royce would today cost $100, get a million miles per gallon, and explode once a year, killing everyone inside.«
– Robert X. Cringely, InfoWorld magazine
Zuletzt bearbeitet von PeaceKiller am 19.01.2006, 21:47, insgesamt einmal bearbeitet |
|
Nach oben |
|
 |
Patrick Dark JLI Master

Anmeldedatum: 25.10.2004 Beiträge: 1895 Wohnort: Düren Medaillen: Keine
|
|
Nach oben |
|
 |
Fallen JLI MVP


Alter: 40 Anmeldedatum: 08.03.2003 Beiträge: 2860 Wohnort: Münster Medaillen: 1 (mehr...)
|
Verfasst am: 19.01.2006, 21:14 Titel: |
|
|
1. Dragon
2. Clythoss
3. Kronos
mehr oder weniger geraten _________________ "I have a Core2Quad at 3.2GHz, 4GB of RAM at 1066 and an Nvidia 8800 GTS 512 on Vista64 and this game runs like ass whereas everything else I own runs like melted butter over a smokin' hot 18 year old catholic schoolgirl's arse." |
|
Nach oben |
|
 |
LordHoto JLI'ler

Alter: 35 Anmeldedatum: 27.03.2003 Beiträge: 137 Wohnort: Gelnhausen Medaillen: Keine
|
Verfasst am: 19.01.2006, 21:16 Titel: |
|
|
Patrick hat Folgendes geschrieben: | Frage am Rande:
CPP: | while(registers[id]); | Unsinn?  |
noe unsichere multithreaded application!
aber sonst sicher bug -> noob code. |
|
Nach oben |
|
 |
KI JLI Master
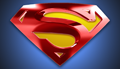
Alter: 39 Anmeldedatum: 04.07.2003 Beiträge: 965 Wohnort: Aachen Medaillen: Keine
|
Verfasst am: 19.01.2006, 22:39 Titel: |
|
|
Ich will meine Statistik in diesem Spiel nicht kaputtmachen.
Aber was solls. Mein Tipp:
1. Dragon
5. Christian Rousselle (aka C.)
bei den anderen weiß ich jetzt nicht. |
|
Nach oben |
|
 |
Kronos Senior JLI'ler

Anmeldedatum: 19.03.2004 Beiträge: 290
Medaillen: Keine
|
Verfasst am: 20.01.2006, 07:50 Titel: |
|
|
1. Christian
2. Dragon
5. Clythoss
Will ja mal was anderes tippen  _________________
David hat Folgendes geschrieben: | Solang meine Beiträge konstruktiver sind als deiner bin ich zufrieden... |
Kein Kommentar notwendig. :rolleyes: |
|
Nach oben |
|
 |
Christian Rousselle Site Admin
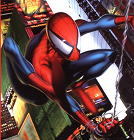
Alter: 48 Anmeldedatum: 19.07.2002 Beiträge: 1630
Medaillen: Keine
|
Verfasst am: 20.01.2006, 08:36 Titel: |
|
|
1. bld
3. Kronos
5. KI |
|
Nach oben |
|
 |
FH Super JLI'ler
Alter: 36 Anmeldedatum: 16.10.2004 Beiträge: 438
Medaillen: Keine
|
Verfasst am: 20.01.2006, 21:58 Titel: |
|
|
Erstmal ne Frage:
Zwischen 1 und 2 ist ein Code ohne Nummer. Zu welchem gehört der denn?
Gruß
FH _________________ goto work, send your kids to school
follow fashion, act normal
walk on the pavement, watch T.V.
save for your old age, obey the law
Repeat after me: I am free |
|
Nach oben |
|
 |
PeaceKiller JLI Master

Alter: 35 Anmeldedatum: 28.11.2002 Beiträge: 970
Medaillen: Keine
|
Verfasst am: 21.01.2006, 00:41 Titel: |
|
|
FH hat Folgendes geschrieben: | Erstmal ne Frage:
Zwischen 1 und 2 ist ein Code ohne Nummer. Zu welchem gehört der denn?
Gruß
FH |
Zu 1. _________________ »If the automobile had followed the same development cycle as the computer, a Rolls-Royce would today cost $100, get a million miles per gallon, and explode once a year, killing everyone inside.«
– Robert X. Cringely, InfoWorld magazine |
|
Nach oben |
|
 |
fast hawk Senior JLI'ler

Anmeldedatum: 15.07.2005 Beiträge: 237 Wohnort: Freiburg Medaillen: Keine
|
Verfasst am: 22.01.2006, 18:09 Titel: |
|
|
<edit>(1. David)</edit>
2. LordHoto
3. ka vielleicht Fallen _________________ Jetziges Projekt: The Ring War
Status: 40%
-----------------------------------
Nicht weil es schwer ist, wagen wir es nicht, sondern weil wir es nicht wagen, ist es schwer.
--
Lucius Annaeus Seneca (4)
röm. Philosoph, Dramatiker und Staatsmann
Zuletzt bearbeitet von fast hawk am 23.01.2006, 11:55, insgesamt einmal bearbeitet |
|
Nach oben |
|
 |
LordHoto JLI'ler

Alter: 35 Anmeldedatum: 27.03.2003 Beiträge: 137 Wohnort: Gelnhausen Medaillen: Keine
|
Verfasst am: 22.01.2006, 20:40 Titel: |
|
|
fast hawk hat Folgendes geschrieben: | 1. David
2. LordHoto
3. ka vielleicht Fallen |
interresant wie du auf mich beim zweiten kommst, wenn du mal das kommentar von Patrick und mir gelesen haettest...
Definition? |
|
Nach oben |
|
 |
David Super JLI'ler
Alter: 39 Anmeldedatum: 13.10.2005 Beiträge: 315
Medaillen: Keine
|
Verfasst am: 22.01.2006, 22:11 Titel: |
|
|
Hi!
Ich hab garnich mitgemacht!
grüße |
|
Nach oben |
|
 |
Fallen JLI MVP


Alter: 40 Anmeldedatum: 08.03.2003 Beiträge: 2860 Wohnort: Münster Medaillen: 1 (mehr...)
|
Verfasst am: 26.01.2006, 17:25 Titel: |
|
|
Auflösung, wir wollen endlich die Auflösung! _________________ "I have a Core2Quad at 3.2GHz, 4GB of RAM at 1066 and an Nvidia 8800 GTS 512 on Vista64 and this game runs like ass whereas everything else I own runs like melted butter over a smokin' hot 18 year old catholic schoolgirl's arse." |
|
Nach oben |
|
 |
Clythoss Junior JLI'ler

Alter: 44 Anmeldedatum: 04.05.2005 Beiträge: 64 Wohnort: Berlin Medaillen: Keine
|
Verfasst am: 27.01.2006, 06:23 Titel: |
|
|
Genau _________________ Derzeitiges Projekt : Wing Fusion
Status: Es geht vorwärts...
-------------------------------------------------------
E=MC²+1W6 |
|
Nach oben |
|
 |
PeaceKiller JLI Master

Alter: 35 Anmeldedatum: 28.11.2002 Beiträge: 970
Medaillen: Keine
|
Verfasst am: 28.01.2006, 12:23 Titel: |
|
|
Ok, dann gibt's jetzt die Auflösung:
- Dragon
- OLiver
- Fast Hawk
- LordHoto
- Christian Rousselle
_________________ »If the automobile had followed the same development cycle as the computer, a Rolls-Royce would today cost $100, get a million miles per gallon, and explode once a year, killing everyone inside.«
– Robert X. Cringely, InfoWorld magazine |
|
Nach oben |
|
 |
|
|
Du kannst keine Beiträge in dieses Forum schreiben. Du kannst auf Beiträge in diesem Forum nicht antworten. Du kannst deine Beiträge in diesem Forum nicht bearbeiten. Du kannst deine Beiträge in diesem Forum nicht löschen. Du kannst an Umfragen in diesem Forum nicht mitmachen.
|
Powered by phpBB © 2001, 2005 phpBB Group Deutsche Übersetzung von phpBB.de
|